Are you gearing up for a Python programming interview? Whether you’re a fresh graduate or an experienced developer looking to switch roles, mastering common Python interview questions and answers is crucial for success. In this comprehensive guide, we’ll dive into a curated list of Python interview questions and answers that cover everything from basic syntax to advanced concepts.
By the time you finish reading, you’ll be well-equipped to tackle even the trickiest Python-related queries that interviewers might throw your way. So, let’s embark on this journey to sharpen your Python skills and boost your confidence for that upcoming interview! Here are the first 25 Python interview questions and answers:
Table of Contents
Beginner Level Python interview questions and answers
Basic Syntax and Data Types:
Q1: What is the difference between a list and a tuple in Python?
A1: Lists are mutable (can be changed) and use square brackets [], while tuples are immutable (cannot be changed) and use parentheses ().
Q2: How do you create a dictionary in Python?
A2: Dictionaries are created using curly braces {} with key-value pairs. For example: my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
Control Flow:
Q3: What is the purpose of the ‘break’ statement in a loop?
A3: The ‘break’ statement is used to exit a loop prematurely, before its normal completion.
Q4: Explain the difference between ‘while’ and ‘for’ loops.
A4: ‘While’ loops repeat as long as a condition is true, while ‘for’ loops iterate over a sequence (like a list or string) or other iterable objects.
Functions:
Q5: What is a lambda function in Python?
A5: A lambda function is a small anonymous function that can have any number of arguments but can only have one expression.
Q6: What is the difference between parameters and arguments in a function?
A6: Parameters are the variables defined in the function declaration, while arguments are the actual values passed to the function when it’s called.
Intermediate Level Python interview questions and answers
Object-Oriented Programming:
Q7: What is inheritance in Python?
A7: Inheritance is a mechanism where a class can inherit attributes and methods from another class.
Q8: Explain the concept of polymorphism in Python.
A8: Polymorphism allows objects of different classes to be treated as objects of a common base class, enabling the same interface to be used for different underlying forms.
Modules and Packages:
Q9: What is the difference between a module and a package in Python?
A9: A module is a single file containing Python code, while a package is a directory containing multiple modules and a special init.py file.
Q10: How do you import a module in Python?
A10: You can import a module using the ‘import’ keyword, like this: import module_name
Exception Handling:
Q11: What is the purpose of the ‘try’ and ‘except’ blocks in Python?
A11: The ‘try’ block is used to enclose code that might raise an exception, while the ‘except’ block handles the exception if it occurs.
Q12: What is the difference between ‘raise’ and ‘assert’ statements?
A12: ‘raise’ is used to explicitly throw an exception, while ‘assert’ is used for debugging purposes to check if a condition is true.
Advanced Level Python interview questions and answers
Decorators and Generators:
Q13: What is a decorator in Python?
A13: A decorator is a design pattern in Python that allows a user to add new functionality to an existing object without modifying its structure.
Q14: Explain what a generator is and how it differs from a regular function.
A14: A generator is a function that returns an iterator object which we can iterate over. It uses ‘yield’ instead of ‘return’ and generates values on-the-fly, saving memory.
Concurrency and Parallelism:
Q15: What is the difference between multithreading and multiprocessing in Python?
A15: Multithreading runs multiple threads within a single process, sharing the same memory space, while multiprocessing runs multiple processes with separate memory spaces.
Q16: What is the Global Interpreter Lock (GIL) in Python?
A16: The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes at once.
Memory Management:
Q17: How does Python manage memory?
A17: Python uses a private heap space to manage memory. It has a built-in garbage collector that recycles all the unused memory.
Q18: What is reference counting in Python?
A18: Reference counting is a technique of storing the number of references or uses of an object. When the reference count drops to zero, the object is deallocated.
Advanced-Data Structures:
Q19: What is a defaultdict in Python?
A19: A defaultdict is a dictionary subclass that calls a factory function to supply missing values, instead of raising a KeyError.
Q20: Explain the difference between deep copy and shallow copy.
A20: A shallow copy creates a new object but references the same memory addresses as the original object for nested objects. A deep copy creates a new object and recursively copies all nested objects.
File I/O:
Q21: How do you read a file in Python?
A21: You can use the ‘open()’ function to open a file and then use methods like ‘read()’, ‘readline()’, or ‘readlines()’ to read its contents.
Q22: What is the difference between ‘r+’ and ‘w+’ modes when opening a file?
A22: ‘r+’ opens a file for both reading and writing but doesn’t truncate the file. ‘w+’ opens a file for both reading and writing and truncates the file if it already exists.
Debugging and Testing:
Q23: What is the purpose of the ‘pdb’ module in Python?
A23: The ‘pdb’ module is Python’s interactive source code debugger. It allows you to pause program execution and examine variables.
Q24: What is unit testing in Python and which module is commonly used for it?
A24: Unit testing is a method of testing individual units of source code. The ‘unittest’ module is commonly used for writing and running tests in Python.
Python Internals:
Q25: What are Python magic methods (or dunder methods)?
A25: Magic methods, also known as dunder methods, are special methods in Python that have double underscores before and after their names. They allow you to emulate the behaviour of built-in types or implement operator overloading.
String Manipulation
Q26: What is string slicing in Python?
A26: String slicing is a way to extract a portion of a string using a range of indices. The syntax is string[start:end:step].
Q27: How do you convert a string to uppercase in Python?
A27: You can use the upper() method, like this: my_string.upper()
List Comprehensions:
Q28: What is a list comprehension in Python?
A28: A list comprehension is a concise way to create lists based on existing lists or other iterable objects. For example: [x**2 for x in range(10)]
Q29: How does a list comprehension differ from a generator expression?
A29: List comprehensions create a full list in memory, while generator expressions yield one item at a time, which is more memory-efficient for large datasets.
Regular Expressions:
Q30: What is the ‘re’ module in Python used for?
A30: The ‘re’ module provides support for regular expressions in Python, allowing pattern matching and manipulation of strings.
Q31: What’s the difference between match() and search() in the ‘re’ module?
A31: match() checks for a match only at the beginning of the string, while search() checks for a match anywhere in the string.
Functional Programming:
Q32: What are the map(), filter(), and reduce() functions in Python?
A32: map() applies a function to all items in an input list, filter() creates a list of elements for which a function returns True, and reduce() applies a function of two arguments cumulatively to the items of a sequence.
Q33: What is a closure in Python?
A33: A closure is a function object that remembers values in enclosing scopes even if they are not present in memory.
Context Managers:
Q34: What is a context manager in Python?
A34: A context manager is an object that defines the runtime context to be established when executing a with statement. It handles the entry into, and the exit from, the desired runtime context.
Q35: How do you create a custom context manager?
A35: You can create a custom context manager by defining a class with enter() and exit() methods, or by using the @contextmanager decorator on a generator function.
Metaclasses:
Q36: What is a metaclass in Python?
A36: A metaclass is a class of a class that defines how a class behaves. It’s the class of a class object and can be used to customize class creation.
Q37: How do you define a metaclass in Python?
A37: You can define a metaclass by creating a class that inherits from ‘type’, and then use it with the metaclass keyword argument in a class definition.
Asynchronous Programming:
Q38: What is asynchronous programming in Python?
A38: Asynchronous programming allows concurrent execution of multiple tasks without using multiple threads, typically using coroutines and the async/await syntax.
Q39: What is the difference between asyncio.gather() and asyncio.wait() in Python?
A39: asyncio.gather() returns results in the order tasks were passed in and raises exceptions, while asyncio.wait() returns completed tasks in the order they finished and doesn’t raise exceptions.
Design Patterns:
Q40: What is the Singleton design pattern and how can it be implemented in Python?
A40: The Singleton pattern restricts the instantiation of a class to one object. It can be implemented using a metaclass or by overriding the new() method.
Q41: Explain the Observer design pattern and give an example in Python.
A41: The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified. It can be implemented using classes with methods for attaching, detaching, and notifying observers.
Performance Optimization:
Q42: What is the purpose of the ‘@lru_cache’ decorator in Python?
A42: @lru_cache is a decorator that implements memoization, caching the results of a function so that if the function is called again with the same arguments, the cached result is returned instead of recomputing it.
Q43: How can you profile a Python script to identify performance bottlenecks?
A43: You can use the cProfile module to profile a Python script. It provides detailed statistics about the time spent in different functions and method calls.
Network Programming:
Q44: What is the ‘socket’ module in Python used for?
A44: The ‘socket’ module provides a way to create network sockets, which are endpoints for sending and receiving data across a computer network.
Q45: How do you create a simple HTTP server in Python?
A45: You can use the http.server module to create a simple HTTP server. For example: python -m http.server 8000 will start a server on port 8000.
Data Science and Machine Learning:
Q46: What are NumPy and Pandas in Python?
A46: NumPy is a library for numerical computing in Python, while Pandas is a data manipulation and analysis library built on top of NumPy.
Q47: What is the difference between a Series and a DataFrame in Pandas?
A47: A Series is a one-dimensional labelled array capable of holding data of any type, while a DataFrame is a two-dimensional labelled data structure with columns of potentially different types.
Web Scraping & Security Python Interview Questions and Answers:
Q48: What is web scraping and which Python libraries can be used for it?
A48: Web scraping is the process of extracting data from websites. Popular Python libraries for web scraping include Beautiful Soup, Scrapy, and Requests.
Q49: How do you handle dynamic content when web scraping?
A49: To handle dynamic content, you might need to use a library like Selenium that can interact with JavaScript-rendered content, or use APIs if available.
Q50: What are some best practices for securely storing passwords in a Python application?
A50: Best practices include using strong hashing algorithms (like bcrypt or Argon2), using salts, and never storing passwords in plain text. The ‘passlib’ library in Python provides secure password hashing.
To Sum Up
As we wrap up our exploration of Python interview questions and answers, it’s clear that being well-prepared can make all the difference in your next tech interview. From fundamental concepts to intricate programming paradigms, we’ve covered a wide spectrum of topics that are likely to come up during your Python-focused discussions with potential employers. Remember, these questions and answers are just the beginning – they serve as a springboard for deeper learning and understanding.
As you continue to practice and expand your Python knowledge, you’ll find yourself not just memorizing answers, but truly grasping the underlying principles that make Python such a powerful and versatile language. Armed with this knowledge and a passion for coding, you’re now ready to step into your Python interview with confidence. Good luck, and may your Python prowess shine through!
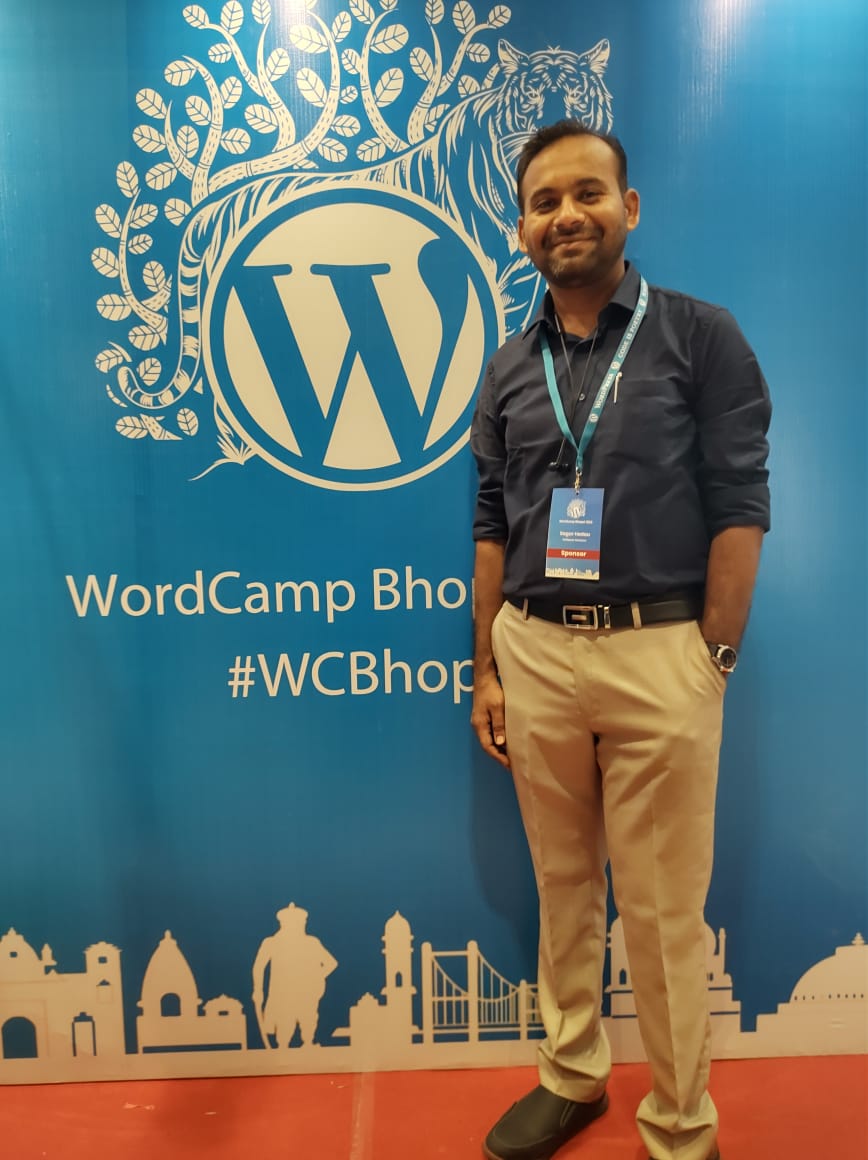
13+ Yrs Experienced Career Counsellor & Skill Development Trainer | Educator | Digital & Content Strategist. Helping freshers and graduates make sound career choices through practical consultation. Guest faculty and Digital Marketing trainer working on building a skill development brand in Softspace Solutions. A passionate writer in core technical topics related to career growth.