Getting ready for a React JS interview? Whether you’re a beginner looking for your first job or an experienced developer looking to switch roles, React JS is one of the most in-demand skills in web development.
Facebook, Netflix and Airbnb use React, so mastering it can get you high-paying job opportunities. But here’s the challenge:
- ✅ React interviews are getting harder.
- ✅ Hiring managers expect you to not only know React but also solve problems.
- ✅ Many candidates fail because of a lack of preparation.
👉 Don’t worry—we’ve got you covered. This article will cover the most common React JS interview questions and answers, from basic to advanced.
So if you want to ace your next React interview, keep reading!
Top 50 React Js Interview Questions And Answers
Q: What is React?
A: React is a popular JavaScript library for building user interfaces, particularly single-page applications. Developed and maintained by Facebook, React allows developers to create reusable UI components that efficiently update and render when data changes. Its declarative approach makes it easier to reason about an application and debug code.
Q: What are the key features of React?
A: Key features of React include:
- Virtual DOM for improved performance
- JSX for writing HTML-like code in JavaScript
- Component-based architecture for reusable UI elements
- Unidirectional data flow for predictable state management
- React hooks for state and lifecycle management in functional components
- Strong community support and a rich ecosystem of libraries
Q: Explain the concept of Virtual DOM.
A: The Virtual DOM is a lightweight, in-memory representation of the actual DOM. When state changes in a React application, it first updates the Virtual DOM, computes the differences (a process called “diffing”), and then efficiently updates only the necessary parts of the actual DOM. This approach significantly improves performance by minimizing expensive DOM manipulation operations.
Q: What is JSX?
A: JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code directly in your JavaScript files. It makes the structure of UI components more readable and expressive. JSX is transformed into regular JavaScript function calls during the build process, typically using tools like Babel.
Q: What are React components?
A: React components are reusable, self-contained pieces of UI that can be composed to build complex user interfaces. They encapsulate both the structure (JSX) and behaviour (JavaScript) of a part of the UI. Components can be either function components or class components, and they can receive props as inputs and return React elements describing what should appear on the screen.
Q: What’s the difference between functional and class components?
A: Functional components are JavaScript functions that accept props and return React elements. They’re simpler, more concise, and since the introduction of hooks, can handle state and side effects. Class components are ES6 classes that extend React.Component. They have their own state, lifecycle methods, and can be more verbose. While both can be used, functional components with hooks are now the preferred approach in modern React development.
Q: Explain React props.
A: Props (short for properties) are a way of passing data from parent to child components in React. They are read-only and help make components reusable. Props can be any JavaScript value type, including functions. When a component’s props change, React re-renders the component. Props follow a unidirectional data flow, meaning data only flows down the component tree.
Q: What is state in React?
A: State in React is an object that holds data that may change over time and affect the component’s rendering. Unlike props, the state is managed within the component (for class components) or with hooks (for functional components). When the state changes, React re-renders the component and its children. State should be considered private to a component and should not be modified directly, but through setState (for class components) or state update functions (for hooks).
Q: What are React hooks?
A: React hooks are functions that allow you to “hook into” React state and lifecycle features from functional components. Introduced in React 16.8, hooks provide a more direct API to the React concepts you already know: props, state, context, refs, and lifecycle. They allow you to use state and other React features without writing a class, making code more readable and easier to test.
Q: Name some commonly used React hooks.
A: Common React hooks include:
- useState: For adding state to functional components
- useEffect: For side effects and lifecycle methods
- useContext: For consuming context
- useReducer: For complex state logic
- useCallback: For memoizing functions
- useMemo: For memoizing values
- useRef: For creating mutable references
- useLayoutEffect: Similar to useEffect, but fires synchronously after all DOM mutations
Q: What is the purpose of useEffect?
A: useEffect is a hook that allows you to perform side effects in functional components. It serves a similar purpose to componentDidMount, componentDidUpdate, and componentWillUnmount in class components, but unified into a single API. useEffect runs after every render by default, but can be optimized to run only when certain values change. It’s commonly used for data fetching, setting up subscriptions, or manually changing the DOM.
Q: How do you handle events in React?
A: React events are named using camelCase and passed as functions rather than strings. They are synthetic events wrapped around the browser’s native events for cross-browser compatibility. Event handlers are typically defined within the component and passed as props to elements. For example:
function handleClick(e) {
e.preventDefault();
console.log('The link was clicked.');
}
return <button onClick={handleClick}>Click me</button>;
Q: What is the significance of keys in React lists?
A: Keys help React identify which items in a list have changed, been added, or been removed. They should be given to the elements inside an array to give them a stable identity. Keys should be unique among siblings but don’t need to be globally unique. Using keys properly helps React update the UI efficiently, improving performance, especially for dynamic lists.
Q: Explain the concept of lifting state up.
A: Lifting state up is a pattern used when several components need to share the same changing data. Instead of trying to sync state between different components, you move the shared state to their closest common ancestor. This makes the state live in a single source of truth, and passes it down to child components that need it. This approach helps avoid bugs caused by the state being out of sync between different components.
Q: What is context in React?
A: Context provides a way to pass data through the component tree without having to pass props down manually at every level. It’s designed to share data that can be considered “global” for a tree of React components, such as the current authenticated user, theme, or preferred language. Context helps avoid “prop drilling” where you pass props through intermediate components that don’t need the data but only pass it along.
Q: What are controlled and uncontrolled components?
A: In a controlled component, form data is handled by the React component’s state. The component renders the form and controls what happens in that form on subsequent user input. An uncontrolled component stores its own state internally, and you query the DOM using a ref to find its current value when you need it. This is more similar to traditional HTML form inputs.
Q: What is React Router?
A: React Router is a standard routing library for React applications. It enables the creation of single-page applications with navigation without the page refreshing as the user navigates. React Router uses component structure to call components, which display the appropriate information. It allows you to structure the URLs of your app in a declarative way, keep your UI in sync with the URL, and handle navigation history.
Q: Difference between React routing and conventional routing?
SN | React Routing | Conventional routing |
1. | It has a Single HTML page | Each view is a new HTML file/page |
2. | The user navigates multiple views in the same file | The user navigates multiple files to get to each view |
3. | Beinga single file, the page does notrefresh | The page refreshes every time to go to new page |
4. | Improves App performance | Slower performance due to multiple pages loading |
Q: How do you optimize performance in React?
A: Performance optimization in React can be achieved through various techniques:
- Use React.memo for functional components to prevent unnecessary re-renders
- Implement shouldComponentUpdate or extend PureComponent for class components
- Use the useMemo hook to memoize expensive computations
- Employ lazy loading with React.lazy and Suspense for code splitting
- Properly use keys in lists to help React identify which items have changed
- Avoid inline function definitions in render methods
- Use production builds for deployment
- Consider using immutable data structures for faster comparisons
Q: What are Higher-Order Components (HOCs)?
A: Higher-Order Components are functions that take a component and return a new component with some additional functionality or props. HOCs are a pattern derived from React’s compositional nature. They’re used for cross-cutting concerns where you want to reuse component logic across multiple components. Examples include adding loading indicators, handling authentication, or injecting data fetching.
Q: Explain the concept of code splitting in React.
A: Code splitting is the practice of splitting your code into smaller chunks which can then be loaded on demand or in parallel. It helps to improve performance by reducing the size of the initial bundle that needs to be downloaded. In React, this can be achieved using dynamic import() syntax along with React.lazy and Suspense. This allows you to load components or modules only when they’re needed, improving the initial load time of your application.
Q: What is the significance of the useState hook?
A: useState is a hook that allows you to add React state to functional components. It returns an array with two elements: the current state value and a function to update it. This eliminates the need for converting function components to classes just to use state. useState can be called multiple times in a single component to manage multiple state variables. It’s particularly useful for local component state.
Q: How do you handle forms in React?
A: Forms in React are typically handled using controlled components. In this approach, form data is stored in the component’s state and updated via onChange events. This gives you more control over the form data and allows for immediate validation and formatting. Here’s a simple example:
function NameForm() {
const [name, setName] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
alert('A name was submitted: ' + name);
}
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={name} onChange={e => setName(e.target.value)} />
</label>
<input type="submit" value="Submit" />
</form>
);
}
Advanced React JS Interview Questions And Answers
Q: What is the purpose of the useCallback hook?
A: useCallback is a hook that returns a memoized version of the callback function that only changes if one of the dependencies has changed. It’s useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders. This can help in performance optimization, especially in scenarios where a component is re-rendering frequently and passing a callback to an expensive child component.
Q: Explain the concept of render props.
A: Render props is a technique for sharing code between React components using a prop whose value is a function. A component with a render prop takes a function that returns a React element and calls it instead of implementing its own render logic. This pattern makes the behaviour that we need to share highly portable. Libraries like React Router and Formik use this pattern.
Q: What are React portals?
A: React portals provide a first-class way to render children into a DOM node that exists outside the DOM hierarchy of the parent component. This is particularly useful for components like modals, tooltips, or floating menus, where you need to break out of the container. Portals can be created using ReactDOM.createPortal(child, container). The typical use-cases for portals are when you need to render a child into a different part of the DOM tree, such as for modals or tooltips.
Q: Explain the concept of React Fiber and its benefits.
A: React Fiber is a complete rewrite of React’s core algorithm. It’s designed to improve the performance of complex React applications by enabling incremental rendering of the virtual DOM. Fiber allows React to pause and resume work, assign priority to different types of updates, and reuse previously completed work. Benefits include improved perceived performance, better scheduling of updates, and smoother animations.
Q: How does React’s reconciliation algorithm work?
A: React’s reconciliation algorithm is the process of updating the DOM with changes made to the React component tree. It works by:
- Comparing the new virtual DOM with the old one
- Identifying the differences (diffing)
Updating only the parts of the actual DOM that have changed This process is efficient because it minimizes expensive DOM operations. React uses heuristics like assuming that elements of different types will produce different trees and using keys to identify which children have changed, been added, or been removed.
Q: What are React Suspense and concurrent mode?
A: React Suspense is a feature that allows components to “wait” for something before rendering, showing a fallback UI in the meantime. It’s particularly useful for handling asynchronous operations like data fetching or code splitting.
Concurrent Mode is a set of new features that help React apps stay responsive and gracefully adjust to the user’s device capabilities and network speed. It allows React to work on multiple tasks concurrently, interrupt and resume work as needed, and abort or deprioritize less important updates.
Q: Describe the useLayoutEffect hook and how it differs from useEffect.
A: useLayoutEffect is similar to useEffect, but it fires synchronously after all DOM mutations. It’s useful when you need to make DOM measurements or updates that should be applied before the browser repaints. The main difference is that useLayoutEffect runs synchronously immediately after React has performed all DOM mutations, while useEffect runs asynchronously after the render is committed to the screen.
Q: What are the main differences between Redux and the Context API for state management?
A: Redux and Context API are both used for state management, but they have some key differences:
- Redux is a full state management library, while Context API is a feature built into React.
- Redux uses a single store for all state, while Context can have multiple, independent contexts.
- Redux is more suitable for complex state logic and large-scale applications, while Context API is simpler and sufficient for many smaller applications.
- Redux provides additional features like middleware, Time Travel Debugging, and a large ecosystem of add-ons.
Q: Difference between Redux & Flux
SN | Redux | Flux |
1. | Redux is an open-source JS library used to manage the State of the application | Flux is not a framework or library. It as an architecture. |
2. | Store’s state is immutable/unchanging | Store’s state is liable to change |
3. | It can only have a single-store | Can have multiple stores |
4. | The concept of reducer is used | The concept of dispatcher is used |
Q: Explain the concept of render props vs. higher-order components.
A: Both render props and higher-order components (HOCs) are patterns for reusing component logic.
Render props involve passing a function as a prop to a component, which the component then calls to render some of its content. This allows for flexible composition and avoids issues with HOCs like prop naming collisions.
HOCs are functions that take a component and return a new component with some additional functionality. They’re useful for cross-cutting concerns but can lead to “wrapper hell” if overused.
Q: How would you implement your own useState hook?
A: A basic implementation of useState might look like this:
let state;
function useState(initialValue) {
state = state || initialValue;
function setState(newValue) {
state = newValue;
render(); // Assume this triggers a re-render
}
return [state, setState];
}
This is a simplified version. React’s actual implementation is more complex, handling multiple state variables and preserving state across re-renders.
Q: What are the best practices for handling side effects in React applications?
A: Best practices for handling side effects include:
- Use the useEffect hook for side effects in functional components
- Clean up side effects when the component unmounts
- Use the dependency array in useEffect to control when effects run
- Separate concerns by using multiple useEffect hooks
- Consider using custom hooks to encapsulate and reuse side-effect logic
- Use async/await for cleaner asynchronous code in effects
- Be mindful of the order of side effects
Q: Describe the process of server-side rendering with React.
A: Server-side rendering (SSR) with React involves:
- Creating a server using Node.js and Express
- Using ReactDOMServer to render React components to HTML strings on the server
- Sending the initial HTML to the client
- Hydrating the application on the client-side with ReactDOM.hydrate()
- Handling routing on both server and client sides
- Managing state consistently between server and client
Libraries like Next.js simplify this process by providing built-in SSR capabilities.
Q: How can you optimize the performance of a React application that renders large lists?
A: Strategies for optimizing large list rendering include:
- Virtualization: Only render items currently in view (react-window or react-virtualized libraries)
- Windowing: Render a small subset of items and “window” the visible content
- Pagination: Split the list into pages and load them on demand
- Infinite scrolling: Load more items as the user scrolls
- Memoization: Use React.memo to prevent unnecessary re-renders of list items
- Proper key usage: Ensure each list item has a unique, stable key
- Code splitting: Lazy load list components if they’re not immediately needed
Q: Explain the concept of lazy loading in React and when you would use it.
A: Lazy loading in React allows you to split your code into smaller chunks and load components only when they’re needed. It’s implemented using React.lazy() and Suspense. You would use lazy loading to:
- Improve initial load time by reducing the initial bundle size
- Load components that are not immediately needed (e.g., components behind a tab or modal)
- Optimize resource usage, especially for large applications Example:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</React.Suspense>
);
}
Q: What are Error Boundaries in React and how do you implement them?
A: Error Boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. They catch errors during rendering, in lifecycle methods, and in constructors of the whole tree below them. To implement an Error Boundary:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
logErrorToMyService(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Q: How would you handle authentication in a React application?
A: Handling authentication in a React application typically involves:
- Creating login/signup forms to collect user credentials
- Sending credentials to a backend server for verification
- Storing authentication tokens (e.g., JWT) in localStorage or secure cookies
- Creating an authentication context to share auth state across the app
- Using higher-order components or custom hooks to protect routes
- Implementing logout functionality to clear tokens and auth state
- Refreshing tokens when they expire
- Handling unauthorized access attempts
Example of a simple auth context:
const AuthContext = React.createContext(null);
function AuthProvider({ children }) {
const [user, setUser] = useState(null);
const login = (userData) => {
setUser(userData);
// Store token in localStorage
};
const logout = () => {
setUser(null);
// Remove token from localStorage
};
return (
<AuthContext.Provider value={{ user, login, logout }}>
{children}
</AuthContext.Provider>
);
}
Q: Describe the differences between useMemo and useCallback hooks.
A: Both useMemo and useCallback are used for optimization, but they serve different purposes:
useMemo:
- Memoizes the result of a computation
- Returns a memoized value
- Recalculates the value only when one of its dependencies changes
- Useful for expensive calculations
useCallback:
- Memoizes a function definition
- Returns a memoized callback function
- Creates a new function only when one of its dependencies changes
- Useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders
Q: What are the challenges of working with forms in React and how do you address them?
A: Challenges with forms in React include:
- Managing form state: Use useState or libraries like Formik
- Handling form submission: Prevent default behaviour and manage async operations
- Validating inputs: Implement custom validation logic or use libraries like Yup
- Handling multiple input types: Create reusable input components
- Performance with large forms: Use techniques like debouncing or throttling
- Accessibility: Ensure proper labelling and keyboard navigation
- File uploads: Handle file input and manage uploads
- Dynamic form fields: Implement logic for adding/removing fields dynamically
Libraries like Formik or react-hook-form can help address many of these challenges.
Q: How would you implement a custom hook for handling API calls?
A: A custom hook for API calls might look like this:
import { useState, useEffect } from 'react';
function useApi(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
async function fetchData() {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
}
fetchData();
}, [url]);
return { data, loading, error };
}
This hook manages the state of an API call, including loading and error states, and can be reused across components.
Q: Explain the concept of prop drilling and strategies to avoid it.
A: Prop drilling occurs when you pass props through multiple levels of components that don’t need those props themselves, just to get them to a deeply nested component. Strategies to avoid prop drilling include:
Follow the Arrange-Act-Assert pattern in your tests
- Using Context API for deeply nested data
- Implementing component composition
- Using state management libraries like Redux
- Creating custom hooks to manage and share state
- Using render props or higher-order components
Q: How does React handle events under the hood?
A: React handles events through a system called Synthetic Events. When you define an event handler in React:
- React attaches a single event listener to the root of the document for each event type.
- When an event occurs, React creates a Synthetic Event object that wraps the native browser event.
- React then dispatches this Synthetic Event to the appropriate components in the virtual DOM tree.
- The Synthetic Event system provides a consistent interface across different browsers.
- Event handlers are bound to instances automatically in class components (when using class fields or arrow functions).
Q: What are the best practices for testing React components?
A: Best practices for testing React components include:
- Use Jest as the test runner and assertion library
- Use React Testing Library or Enzyme for rendering and interacting with components
- Write unit tests for individual components
- Write integration tests for component interactions
- Use snapshot testing for UI components
- Mock API calls and complex dependencies
- Test edge cases and error scenarios
- Use code coverage tools to ensure adequate test coverage
- Implement continuous integration to run tests automatically
Q: How would you implement a debounce function in React?
A: A debounce function delays the processing of a function until after a certain amount of time has passed since the last time it was invoked. Here’s an example of a custom hook implementing debounce:
import { useState, useEffect } from 'react';
function useDebounce(value, delay) {
const [debouncedValue, setDebouncedValue] = useState(value);
useEffect(() => {
const handler = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue;
}
This hook can be used to debounce input values, search queries, or any other frequently changing value.
Q: Explain the concept of React portals and their use cases.
A: React portals provide a way to render children into a DOM node that exists outside the DOM hierarchy of the parent component. The syntax is:
ReactDOM.createPortal(child, container)
Use cases include:
- Modals and dialogs that need to break out of the parent container
- Tooltips or popovers that need to overlay other content
- Floating menus
- Widgets that need to be rendered at a specific DOM location
Portals are useful when you need to render content outside of the normal component tree structure while still maintaining the React context.
Q: How does React’s diffing algorithm work?
A: React’s diffing algorithm, also known as reconciliation, works as follows:
- When a component’s props or state changes, React creates a new virtual DOM tree.
- React then compares this new tree with the previous one.
- It starts at the root and works its way down, comparing each node:
- If the element types are different, it assumes the entire subtree has changed.
- For elements of the same type, it updates the props.
- For components, it calls the render method and repeats the process for the rendered elements.
- When comparing lists of children, React uses keys to identify which items have changed, been added, or been removed.
- Once the diffing is complete, React knows exactly which parts of the actual DOM need to be updated and makes these changes in a single pass.
This algorithm has a complexity of O(n) where n is the number of elements in the tree, making it very efficient for most use cases. When a component’s props or state changes, React creates a new virtual DOM tree.
Q: What are the advantages and disadvantages of using CSS-in-JS libraries with React?
A: Advantages of CSS-in-JS:
- Scoped styles: Styles are automatically scoped to components
- Dynamic styling: Can easily create styles based on props or state
- Dead code elimination: Unused styles can be automatically removed
- Sharing variables: Easy to share variables between JS and CSS
- Automatic vendor prefixing: Many libraries handle this automatically
Disadvantages:
- Learning curve: Requires learning a new API and way of thinking about styles
- Performance overhead: Can introduce a small runtime performance cost
- Increased bundle size: Adds additional JavaScript to your bundle
- Lack of caching: Styles are typically not cached separately from JavaScript
- Potential for overuse: This can lead to inline styles and harder-to-maintain code if not used carefully
- Q: How would you implement an infinite scroll in React? A: To implement infinite scroll:
- Use a library like react-window or react-virtualized for efficient rendering of large lists
- Implement a scroll event listener or use the Intersection Observer API
- When the user scrolls near the bottom, trigger a function to load more data
- Update the state with the new data, which will cause React to re-render
- Optionally, show a loading indicator while fetching data
Here’s a basic example using the Intersection Observer API:
import React, { useState, useRef, useCallback } from 'react';
function InfiniteScroll({ loadMore }) {
const [items, setItems] = useState([]);
const observer = useRef();
const lastItemRef = useCallback(node => {
if (observer.current) observer.current.disconnect();
observer.current = new IntersectionObserver(entries => {
if (entries[0].isIntersecting) {
loadMore().then(newItems => setItems(prev => [...prev, ...newItems]));
}
});
if (node) observer.current.observe(node);
}, [loadMore]);
return (
<div>
{items.map((item, index) => (
<div key={item.id} ref={index === items.length - 1 ? lastItemRef : null}>
{item.content}
</div>
))}
</div>
);
}
Q: Explain the concept of component composition
A: Component composition in React is the practice of building complex user interfaces by combining simpler, reusable components. Instead of creating large, monolithic components, we break them down into smaller, manageable pieces that can be composed together.
Key aspects:
- It favours composition over inheritance for component relationships
- It uses smaller components as building blocks for larger ones.
- It often utilizes the ‘children’ prop for flexible content insertion.
function Card({ children }) {
return <div className="card">{children}</div>;
}
function App() {
return (
<Card>
<h2>Title</h2>
<p>Content</p>
</Card>
);
}
Benefits:
- Improved code reusability and maintainability
- Enhanced flexibility in UI structure
- Better separation of concerns
- Easier testing of individual components
- Facilitates collaboration in team environments
Component composition is a core principle in React, promoting modular, scalable, and maintainable code structures.
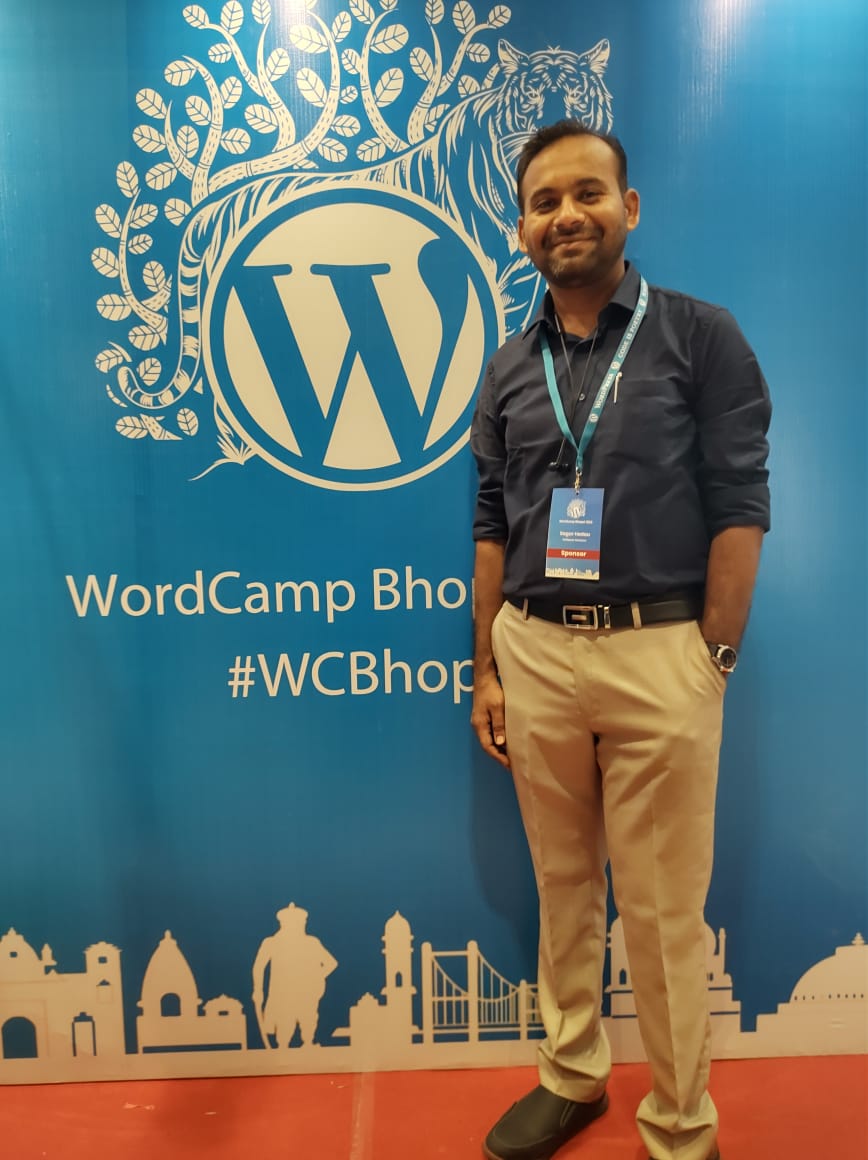
13+ Yrs Experienced Career Counsellor & Skill Development Trainer | Educator | Digital & Content Strategist. Helping freshers and graduates make sound career choices through practical consultation. Guest faculty and Digital Marketing trainer working on building a skill development brand in Softspace Solutions. A passionate writer in core technical topics related to career growth.