Are you gearing up for a Node.js developer interview? Whether you’re a seasoned pro or just starting your journey in server-side JavaScript, being well-prepared is key to landing your dream job. In today’s fast-paced tech world, Node.js continues to be a powerhouse for building scalable and efficient web applications. As its popularity soars, so does the competition for Node.js developer positions.
That’s why we’ve compiled this comprehensive guide to Nodejs interview questions and answers. From core concepts to advanced topics, we’ll cover everything you need to know to ace your next interview. We’ll explore event-driven architecture, asynchronous programming, streams, and much more. Whether you’re facing a technical screening or an in-depth coding challenge, this blog post will equip you with the knowledge to tackle even the toughest Nodejs interview questions and answers.
So, let’s dive in and unlock the secrets to impressing your future employers with good preparation on Nodejs interview questions and answers.
NodeJS Interview Questions And Answers
Core Node.js Concepts
Core Node.js concepts are essential for any developer working with this technology. At its heart, Node.js uses an event-driven, non-blocking I/O model, which allows it to handle many operations concurrently despite being single-threaded. The Event Loop is central to this architecture, managing the execution of code and handling asynchronous operations efficiently.
Node.js leverages Buffers for handling binary data and Streams for efficiently processing data in chunks. Its module system allows for code organization and reusability, while global objects like ‘global’, ‘process’, and ‘console’ provide important functionalities. Understanding asynchronous programming, including callbacks, Promises, and async/await, is crucial for writing efficient Node.js applications.
Proper error handling and file system operations round out the core concepts that any Node.js developer should master. These foundational elements form the backbone of Node.js development and are often the focus of technical interviews.
Q1: What is Node.js?
A1: Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside a web browser. It allows developers to use JavaScript to write command-line tools and for server-side scripting.
Q2: Explain the event-driven architecture in Node.js.
A2: Node.js uses an event-driven, non-blocking I/O model. This means it can handle many connections concurrently. Upon each connection, the callback is fired, but if there’s no work to be done, Node.js will sleep.
Q3: What is the difference between synchronous and asynchronous functions in Node.js?
A3: Synchronous functions block the program’s execution until it is complete, while asynchronous functions allow the program to continue running while it executes in the background, using callbacks or promises to handle the results.
Q4: What is the Event Loop in Node.js?
A4: The Event Loop is what allows Node.js to perform non-blocking I/O operations despite JavaScript being single-threaded. It handles the execution of multiple chunks of your program over time, each time invoking the appropriate event handlers.
Q5: What is the purpose of module.exports?
A5: module.exports is used to export functions, objects, or primitives from a given file so that other files are allowed to access them using the require() function.
Q6: What is the difference between process.nextTick() and setImmediate()?
A6: process.nextTick() defers the execution of an action till the next pass around the event loop or it simply calls the callback function once the ongoing execution of the event loop is finished. setImmediate() executes a callback on the next cycle of the event loop.
Q7: What are Buffers in Node.js?
A7: Buffers are used to store raw binary data and to handle streams of binary data. Buffers act somewhat like arrays of integers but aren’t resizable and have a whole bunch of methods specifically for binary data.
Q8: What is the purpose of the Buffer class in Node.js?
A8: The Buffer class in Node.js is used to handle binary data. It provides a way of handling streams of binary data and performing operations on that data.
Q9: What is the difference between Node.js and JavaScript?
A9: JavaScript is a programming language, while Node.js is a runtime environment for executing JavaScript outside of a browser. Node.js adds functionalities like file system I/O, networking, etc., which aren’t available in browser-based JavaScript.
Q10: What is middleware in Node.js?
A10: Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle, usually denoted by a variable named next.
Looking for super Node.JS hosting?
AccuWeb.Cloud is the best for Node.js hosting due to its optimized environment, high performance with SSD storage, 99.9% uptime, and developer-friendly features like one-click deployment and SSH access.
It ensures robust security with free SSL, DDoS protection, and automated backups. Affordable pricing, 24/7 expert support, and versatile database compatibility make it ideal for businesses and developers seeking reliable and scalable hosting.
1. Optimized Environment for Node.js
- Pre-configured for Node.js: Servers are optimized specifically to handle the asynchronous, event-driven architecture of Node.js.
- Latest Versions: Easy access to the latest versions of Node.js, ensuring compatibility with modern libraries and frameworks.
2. High Performance
- Fast SSD Storage: Ensures rapid read/write speeds for faster application performance.
- Low Latency Servers: Strategically placed data centers reduce latency and ensure quick response times.
- Resource Scalability: Seamless scaling to handle traffic spikes without compromising performance.
3. Reliable Uptime
- 99.9% Uptime Guarantee: Ensures your applications remain accessible around the clock.
- Redundant Infrastructure: Minimizes downtime risk with robust backup systems.
4. Developer-Friendly Features
- One-Click Deployment: Simplifies setting up Node.js apps with minimal effort.
- SSH Access: Full control for advanced configuration and development.
- Support for NPM and Modules: Hassle-free installation and management of Node.js packages.
5. Security
- Free SSL Certificates: Enhances application security with HTTPS encryption.
- Regular Backups: Protects against data loss with automated backup solutions.
- DDoS Protection: Safeguards applications from malicious attacks.
Node.js Modules
Node.js modules are a fundamental concept in the Node.js ecosystem. They allow developers to organize code into reusable components, making applications more maintainable and scalable. At its core, a module is simply a JavaScript file that encapsulates related code. Node.js uses the CommonJS module system by default, though it also supports ECMAScript (ES) modules.
The ‘require()’ function is used to import modules, while ‘module.exports’ or ‘exports’ is used to expose functionality from a module. Node.js comes with several built-in core modules like ‘fs’ for file system operations, ‘http’ for creating HTTP servers, and ‘path’ for handling file paths. Additionally, developers can create their own custom modules or use third-party modules from the npm registry.
Understanding how to create, import, and use modules effectively is crucial for writing clean, modular Node.js code. This knowledge is often tested in interviews, as it’s a key aspect of Node.js development.
Q11: What is a module in Node.js?
A11: A module in Node.js is a reusable block of code whose existence does not accidentally impact other code. Node.js has a simple module loading system that uses the require
function to load modules.
Q12: How do you create a custom module in Node.js?
A12: You can create a custom module by defining properties or methods on the exports
object or by assigning an entire object to module.exports
.
Q13: What’s the difference between module.exports and exports?
A13: exports is a reference to module.exports. If you assign directly to exports, it will no longer reference module.exports. module.exports is the object that’s actually returned as the result of a require() call.
Q14: How does the require function work in Node.js?
A14: The require function in Node.js is used to import modules. It reads a JavaScript file, executes it, and then returns the exports object.
Q15: What are the core modules in Node.js?
A15: Core modules are the fundamental modules that are part of Node.js and don’t need to be installed separately. Examples include ‘fs’ for file system operations, ‘http’ for HTTP operations, ‘path’ for handling file paths, etc.
Node.js Package Management
Node.js package management is primarily centered around npm (Node Package Manager), which comes bundled with Node.js installations. npm is both a command-line tool and an online repository of JavaScript packages. It plays a crucial role in the Node.js ecosystem by allowing developers to easily share and reuse code.
The core of npm functionality revolves around the package.json file, which serves as a manifest for your project. This file contains metadata about the project, including its name, version, dependencies, and scripts. npm uses this file to manage project dependencies and scripts.
Key aspects of Node.js package management include:
- Installing packages: Use ‘npm install’ to add packages to your project.
- Managing dependencies: Distinguish between regular dependencies and devDependencies.
- Semantic versioning: Understand how npm uses semver for package versions.
- Scripts: Utilize npm scripts for automating tasks.
- Publishing packages: Know how to share your own packages on the npm registry.
Alternative package managers like Yarn have also gained popularity, offering features like faster installation and guaranteed dependency versions. Understanding package management is crucial for efficient Node.js development, as it enables developers to leverage the vast ecosystem of open-source packages and tools available. In interviews, candidates are often expected to demonstrate familiarity with npm commands, package.json structure, and best practices for managing project dependencies.
Q16: What is npm?
A16: npm (Node Package Manager) is the default package manager for Node.js. It consists of a command line client and an online database of public and paid-for private packages, called the npm registry.
Q17: What is the purpose of package.json file?
A17: The package.json file holds various metadata relevant to the project. This file is used to give information to npm that allows it to identify the project as well as handle the project’s dependencies.
Q18: What’s the difference between dependencies and devDependencies in package.json?
A18: dependencies are packages required for the application to run, while devDependencies are packages that are only needed for local development and testing.
Q19: What is the purpose of the npm install command?
A19: The npm install command is used to install all dependencies listed in the package.json file. If a package name is provided, it installs that specific package.
Q20: What is yarn? How is it different from npm?
A20: Yarn is an alternative package manager to npm. It was created to address some of npm’s shortcomings, like speed and consistency. Yarn uses a lockfile to ensure consistent installs across machines.
Asynchronous Programming in Node.js
Asynchronous programming is a cornerstone of Node.js, enabling it to handle multiple operations concurrently without blocking the execution thread. This approach is crucial for building scalable and efficient applications, especially when dealing with I/O operations.
In interviews, candidates might be asked to explain these concepts, demonstrate how to convert callback-based code to Promises or async/await, or solve problems that require asynchronous handling. It’s also important to understand common pitfalls like callback hell and how to avoid them.
Q21: Explain callback hell and how to avoid it.
A21: Callback hell refers to heavily nested callbacks that make code hard to read and maintain. It can be avoided by using Promises, async/await, or by modularizing the code.
Q22: What are Promises in Node.js?
A22: Promises are objects representing the eventual completion or failure of an asynchronous operation. They provide a way to handle asynchronous operations without deep nesting of callbacks.
Q23: What is async/await in Node.js?
A23: async/await is a way to write asynchronous code that looks and behaves more like synchronous code. It’s built on top of Promises and is particularly useful for managing complex asynchronous operations.
Q24: How does async/await work in Node.js?
A24: The async keyword is used to define an asynchronous function that returns a Promise. The await keyword can only be used inside an async function, and it waits for a Promise to resolve before continuing execution.
Q25: What is the purpose of the util.promisify() function?
A25: util.promisify() is used to convert a callback-based function to a Promise-based one. This allows you to use older Node.js APIs that use callbacks with the more modern async/await syntax.
Q26: What is the difference between setImmediate() and setTimeout()?
A26: setImmediate() is designed to execute a script once the current poll phase completes, while setTimeout() schedules a script to be run after a minimum threshold in ms has elapsed.
Q27: What is the purpose of process.nextTick()?
A27: process.nextTick() defers the execution of a function until the next pass around the event loop. It’s not technically part of the event loop, but rather a part of the Node.js process itself.
Q28: How does Node.js handle child threads?
A28: While Node.js is single-threaded, it can spawn child processes using the child_process module, or create worker threads using the worker_threads module for CPU-intensive tasks.
Q29: What is the purpose of the cluster module in Node.js?
A29: The cluster module allows you to create child processes that run simultaneously and share the same server port. It’s used to take advantage of multi-core systems to handle load.
Q30: What are Event Emitters in Node.js?
A30: Event Emitters are a core concept in Node.js. Many objects in Node emit events. These objects are instances of the EventEmitter class. It allows us to create and handle custom events.
Node.js Streams
Node.js streams are a powerful and efficient way to handle reading and writing data. They are particularly useful when working with large amounts of data or when data is coming from an external source piece by piece. Streams allow you to process data in chunks as it arrives, rather than waiting for the entire data set to be available.
In interviews, you might be asked to explain how streams work, and their advantages over other data-handling methods, or to solve problems that involve reading, writing, or transforming data using streams. You might also be asked about error handling in streams or how to implement custom streams.
Q31: What are streams in Node.js?
A31: Streams are objects that let you read data from a source or write data to a destination in continuous fashion. There are four types of streams: Readable, Writable, Duplex, and Transform streams.
Q32: What are the different types of streams in Node.js?
A32: There are four fundamental stream types in Node.js: Readable (for reading), Writable (for writing), Duplex (for both reading and writing), and Transform (for modifying data as it’s written and read).
Q33: How do you handle stream events in Node.js?
A33: Stream events can be handled using the on() method. Common events include ‘data’, ‘end’, ‘error’, and ‘finish’.
Q34: What is piping in Node.js streams?
A34: Piping is a mechanism to connect the output of one stream to the input of another stream. It’s done using the pipe() method.
Q35: What is the purpose of the stream.pipe() method?
A35: The stream.pipe() method is used to attach a Writable stream to a Readable stream, causing the push automatically, so that the flow-control is handled automatically.
Error Handling in Node.js
Q36: How do you handle errors in Node.js?
A36: Errors can be handled using try-catch blocks for synchronous code, and through error-first callbacks or .catch() methods on Promises for asynchronous code.
Q37: What is the purpose of the uncaughtException event?
A37: The uncaughtException event is emitted when an uncaught JavaScript exception bubbles all the way back to the event loop. It’s a way to prevent the Node.js process from crashing when an unhandled exception occurs.
Q38: How can you create custom errors in Node.js?
A38: You can create custom errors by extending the built-in Error class. This allows you to add custom properties and methods to your error objects.
Q39: What is error-first callback in Node.js?
A39: Error-first callback is a convention used in Node.js whereby the first argument to the callback function is reserved for an error object. If an error occurs, it will be passed as the first argument. If no error occurs, the first argument will be null.
Q40: How does Node.js handle unhandled promise rejections?
A40: Node.js emits an ‘unhandledRejection’ event when a Promise is rejected and no error handler is attached to it. As of Node.js 15, unhandled promise rejections will terminate the Node.js process.
Node.js and Databases
Q41: How can Node.js connect with MongoDB?
A41: Node.js can connect to MongoDB using the official MongoDB driver for Node.js or an ODM (Object Document Mapper) like Mongoose.
Q42: What is an ORM? Can you name an ORM for Node.js?
A42: ORM stands for Object-Relational Mapping. It’s a technique that lets you query and manipulate data from a database using an object-oriented paradigm. Sequelize is a popular ORM for Node.js that supports multiple SQL databases.
Q43: How do you handle database migrations in Node.js?
A43: Database migrations in Node.js can be handled using tools like Knex.js or Sequelize CLI. These tools allow you to write migration scripts to update your database schema over time.
Q44: What is the purpose of the mongoose.connect() function?
A44: mongoose.connect() is used to establish a connection between your Node.js application and a MongoDB database. It takes a connection string as an argument and returns a promise.
Q45: How can you ensure database queries don’t block the event loop?
A45: To prevent database queries from blocking the event loop, you should use asynchronous methods provided by your database driver or ORM. These methods typically return Promises or accept callbacks.
Node.js Security
Q46: What are some security best practices in Node.js?
A46: Some security best practices include: using HTTPS, implementing proper authentication and authorization, validating user inputs, keeping dependencies updated, and using security headers.
Q47: How can you prevent SQL injection in Node.js?
A47: SQL injection can be prevented by using parameterized queries or prepared statements, and by validating and sanitizing user inputs. ORMs like Sequelize also protect against SQL injection.
Q48: What is the purpose of the helmet middleware in Express.js?
A48: Helmet helps secure Express apps by setting various HTTP headers. It’s not a silver bullet, but it can help protect your app from some well-known web vulnerabilities.
Q49: How can you implement rate limiting in a Node.js application?
A49: Rate limiting can be implemented using middleware like express-rate-limit. This helps prevent abuse of your API by limiting the number of requests a user can make in a given time period.
Q50: What is CSRF and how can you prevent it in Node.js?
A50: CSRF (Cross-Site Request Forgery) is an attack that forces a user to execute unwanted actions on a web application in which they’re authenticated. It can be prevented using the csurf middleware in Express.js, which requires a CSRF token for requests that could modify data.
Some More Important Nodejs Interview Questions and Answers
Q51: How does Node.js work?
A51: The simple process flow of NodeJS working can be worked out as follows:
- Client Requests Arrive: Clients (browsers, apps) send requests to interact with the web application (data retrieval, updates, etc.).
- Event Queue Holds Requests: Node.js receives these requests and queues them in the Event Queue for processing.
- Event Loop Manages Flow: The single-threaded Event Loop continuously monitors the queue.
- Simple Requests Get Handled Quickly: The Event Loop processes simple requests (no external resources) using techniques like I/O Polling and sends responses directly.
- Thread Pool Takes on Complex Tasks: For requests requiring external resources (databases, filesystems, etc.), the Event Loop assigns a thread from the Thread Pool.
- Threads Complete Blocking Operations: The dedicated thread executes the blocking task (e.g., database access) and gathers the required data.
- Response Assembled and Sent: Once complete (by Event Loop or thread), the response is assembled and sent back to the client.
- Efficient and Scalable: This non-blocking approach allows Node.js to handle many concurrent requests efficiently and scale based on server resources.
Q52: Why is Node.js single-threaded?
A52: Node.js is single-threaded for three key reasons:
- Simplicity: Single-threaded code avoids the complexity of managing multiple threads and potential race conditions.
- Non-Blocking I/O: Node.js shines in I/O bound tasks. A single thread with non-blocking I/O efficiently handles many connections without getting stuck on any one request.
- Event Loop Power: The Event Loop efficiently handles simple requests and complex tasks via the Thread Pool, maintaining responsiveness under high load.
While single-threaded, Node.js utilizes a Thread Pool for CPU-intensive tasks and offers features like Web Workers for more complex background processing. This design keeps code simple, efficient, and scalable for modern web applications.
Q52: How does Node.js handle concurrency?
A53: Even though Node.js is single-threaded, it cleverly handles concurrency through a combination of techniques:
- Non-Blocking I/O: Node.js excels at handling tasks that involve waiting for external resources (like databases). It doesn’t block the main thread while waiting; instead, it uses non-blocking I/O techniques to check for available data efficiently.
- Event Loop: The single-threaded Event Loop acts like a traffic controller. It continuously monitors an Event Queue where incoming requests are placed. The Event Loop processes simple requests (not requiring external resources) quickly and sends responses.
- Thread Pool: For complex requests that need external resources, the Event Loop delegates the task to a thread from the Thread Pool. These threads handle the blocking operations (like database access) without impacting the main thread.
- Callbacks and Promises: Node.js uses callbacks or promises to handle asynchronous operations. These mechanisms allow the Event Loop to move on to other tasks while waiting for the blocking operation to finish. Once the thread completes the task, it notifies the Event Loop through the callback or promise, and the response is sent back.
In essence, while Node.js itself is single-threaded, it utilizes an asynchronous architecture with non-blocking I/O, the Event Loop, the Thread Pool, and callbacks/promises to efficiently manage concurrent requests. This allows it to be highly responsive and handle many connections simultaneously.
Q53: What is REPL in Node.js?
A53: REPL, in Node.js, stands for Read-Eval-Print Loop. It’s an interactive shell environment built into Node.js that allows you to execute JavaScript code directly.
Here’s how REPL works:
- Loop: It waits for you to enter another line of code, and the cycle repeats.
- Read: It reads the JavaScript code you enter line by line.
- Evaluate: It evaluates the code and interprets it as JavaScript instructions.
- Print: It prints the result of the evaluation to the console.
Q54: Why does Google use the V8 engine for Node.JS?
A54: For the following reasons related to performance boosting:
- Performance: V8’s speed is ideal for Node.js’s fast request handling.
- Open Source: Both V8 and Node.js benefit from being open-source.
- Shared Expertise: Google’s involvement in both projects ensured compatibility.
- JavaScript Everywhere: V8 enabled Node.js’s core idea of server-side JavaScript.
- Non-Blocking Fit: V8’s design works well with Node.js’s asynchronous approach.
Overall, V8 offered a high-performing, open-source foundation for Node.js, promoting server-side JavaScript development.
Q55: What is WASI?
WASI in Node.js lets you run WebAssembly (Wasm) modules securely:
- Standard Access: WASI provides a consistent way for Wasm modules to access resources in Node.js.
- Security Boost: WASI enforces stricter security compared to traditional methods.
- Focused Use Cases: WASI with Wasm isn’t a replacement for everything, but it’s useful for:
- Running potentially untrusted code (with limitations) securely.
- Integrating performant Wasm modules for specific tasks.
WASI in Node.js is new but holds promise for secure and portable Wasm alongside Node.js code.
Q56: What is the difference between Node.js and Ajax?
A56: Node.js vs. Ajax:
- Node.js: Server-side: Runs on the server, handles data and builds applications.
- Ajax: Client-side: Runs in the browser, and fetches data from the server for a smoother user experience (no full page reloads).
Think of it like a restaurant:
- Node.js: The kitchen (prepares data and responses).
- Ajax: The waiter (takes your order and brings food without needing you to get up).
They work together: Node.js powers the backend, and Ajax enhances the user experience by fetching data from the server.
Q57: Why keep Express App and Server separate?
A57: Here’s why separating the Express app and server in Node.js is a good practice:
- Improved Organization: Separating concerns keeps your code cleaner and more maintainable. The
app.js
file focuses on application logic (routes, middleware), whileserver.js
handles server setup (port, error handling). - Testing Benefits: You can test the Express app (API logic) in isolation without involving the server or network calls. This allows for faster and more focused unit testing.
- Deployment Flexibility: The server configuration (port, environment variables) can be managed separately, making deployment to different environments easier. The app logic remains unchanged.
- Scalability: If you need to scale your application, you can potentially scale the server (processes) independently of the app logic.
Here’s a quick analogy: Separating the app and server is like separating the engine and controls of a car. You can modify the controls (app) without needing to rebuild the engine (server) every time.
To Conclude
To conclude our comprehensive guide on Nodejs interview questions and answers, let’s recap the key takeaways:
We’ve covered a wide range of topics essential for any Node.js developer, from core concepts like the event-driven architecture and the Event Loop, to more advanced subjects like streams, asynchronous programming, and security best practices. By mastering these areas, you’ll be well-equipped to tackle even the most challenging interview questions.
Remember, the goal of these interviews isn’t just to test your memorization skills, but to assess your understanding of Node.js principles and your ability to apply them in real-world scenarios. As you prepare, focus on not just knowing the answers, but understanding the underlying concepts and their practical applications.
Keep in mind that the Node.js ecosystem is constantly evolving. Stay curious and continue learning even after your interview. Follow Node.js releases, explore new packages, and experiment with different coding patterns to keep your skills sharp.
Lastly, don’t forget the importance of soft skills. Technical knowledge is crucial, but so is your ability to communicate clearly, work in a team, and approach problems creatively. Be prepared to discuss your experiences, the challenges you’ve overcome, and your passion for Node.js development.
With thorough preparation and the knowledge you’ve gained from this guide, you’re now ready to confidently approach your Node.js interview. Good luck, and may your Node.js career flourish!
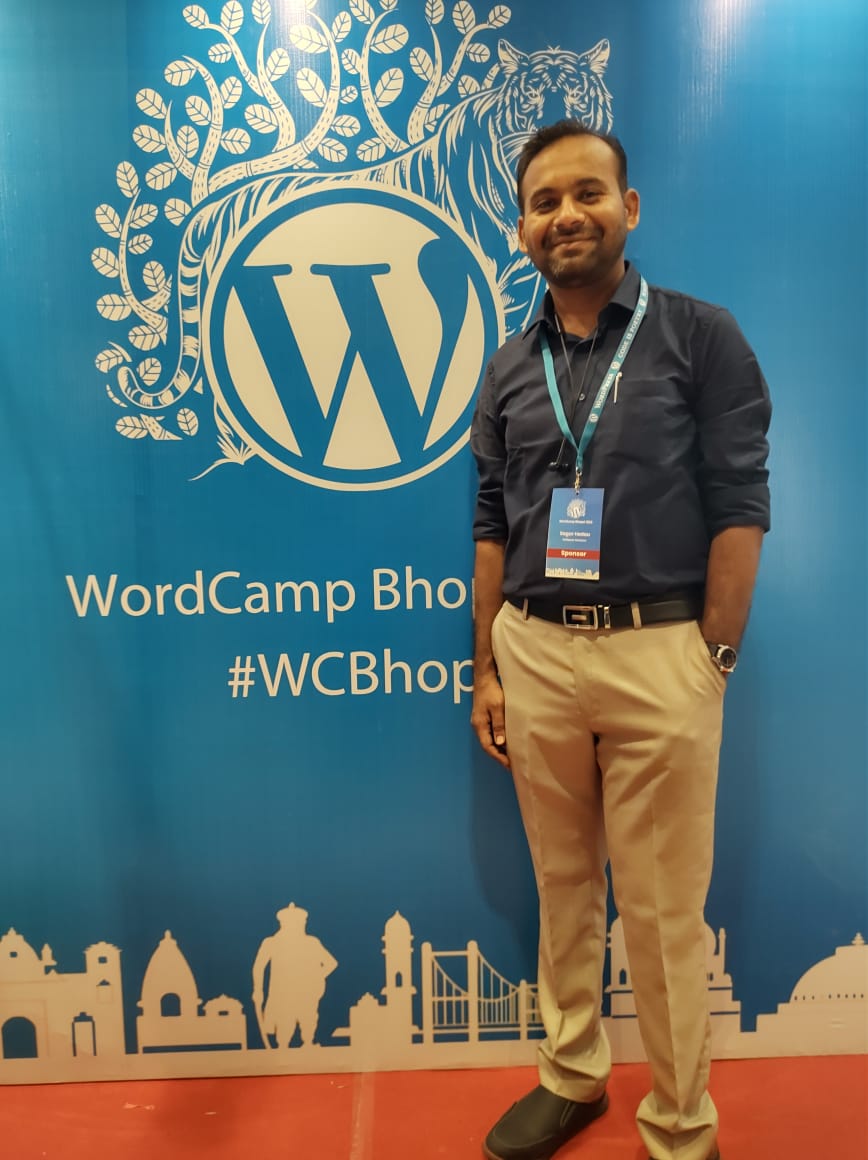
13+ Yrs Experienced Career Counsellor & Skill Development Trainer | Educator | Digital & Content Strategist. Helping freshers and graduates make sound career choices through practical consultation. Guest faculty and Digital Marketing trainer working on building a skill development brand in Softspace Solutions. A passionate writer in core technical topics related to career growth.